6. Data Model Converter Object
A data model converter provides the ability to convert data between a data model and a specific data source or format, such as the PSS/E RAW format or version 2 of the MATPOWER case format. It is used, for example, during the import stage of the data model build process.
6.1. Data Model Converters
A data model converter object is primarily a container for data model converter element objects. All data model converter classes inherit from mp.dm_converter
and therefore also from mp.element_container
and they are specific to the type or format of the data source, as shown in Figure 6.1. In this example, the PSS/E RAW format converter has not yet been implemented, but is shown here for illustration.
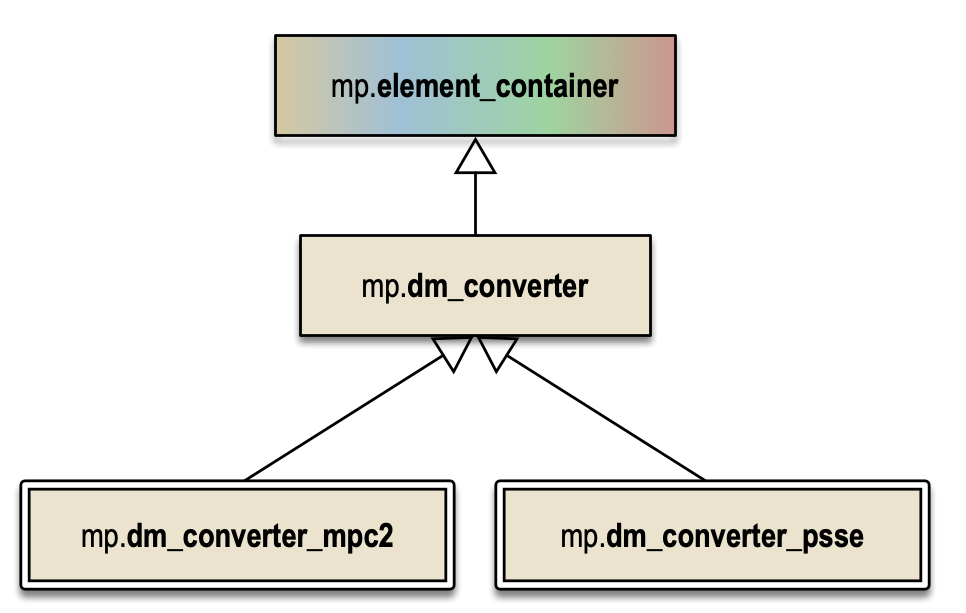
Figure 6.1 Data Model Converter Classes
By convention, data model converter variables are named dmc
and data model converter class names begin with mp.dm_converter
.
6.1.1. Building a Data Model Converter
A data model converter object is created in two steps. The first is to call the constructor of the desired data model converter class, without arguments. This initializes the element_classes
property with a list of data model converter element classes. This list can be modified before the second step, which is to call the build()
method, also without parameters, which simply instantiates and adds the set of element objects indicated in element_classes
. Once it has been created, it is ready to be used for its two primary functions, namely import and export.
dmc = mp.dm_converter_mpc2();
dmc.build();
6.1.2. Importing Data
The import()
method is called automatically by the build()
method of the data model object. It takes a data model object and a data source and updates the data model by looping through its element objects and calling each element’s own import()
method to import the element’s data from the data source into the corresponding data model element. For a MATPOWER case struct it would like like this.
mpc = loadcase('case9');
dm = dmc.import(dm, mpc);
6.1.3. Exporting Data
Conversely, the export()
method takes the same inputs but returns an updated data source, once again looping through its element objects and calling each element’s own export()
method to export data from the corresponding data model element to the respective portion of the data source.
mpc = dmc.export(dm, mpc);
Calling export()
without passing in a data source will initialize one from scratch.
mpc = dmc.export(dm);
6.2. Data Model Converter Elements
A data model converter element object implements the functionality needed to import and export a particular element type from and to a given data format. All data model converter element classes inherit from mp.dmc_element
and each element type typically implements its own subclass.
By convention, data model converter element variables are named dmce
and data model converter element class names begin with mp.dmce
. Figure 6.1 shows the inheritance relationships between a few example data model converter element classes. Here the PSS/E classes have not yet been implemented, but are shown here for illustration.

Figure 6.2 Data Model Converter Element Classes
6.2.1. Data Import Specifications
The default import()
method for a data model converter element first calls the get_import_spec()
method to get a struct containing the specifications that define the details of the import process. This specification is then passed to import_table_values()
to import the data.
The import specifications include things like where to find the data in the data source, the number of rows, number of columns, and possibly a row index vector for rows of interest, [1] and a map defining how to import each column of the main data table.
This map vmap
is a struct returned by the table_var_map()
method with fields matching the table column names for the corresponding data model element dme
. For example, if vn
contains a variable, that is column, name, then vmap.(vn) = <value>
defines how that data table column will be imported or initialized, as summarized in Table 6.1 for different types of values.
|
Description |
---|---|
|
Assign consecutive IDs starting at 1. |
|
Copy the data directly from column |
|
Create a cell array with each element initialized with |
|
Create a numeric vector with each element initialized with numeric scalar |
|
Assign the values returned by the import function handle in |
The table_var_map()
in mp.dmc_element
initializes each entry to {'col', []}
by default, so subclasses only need to assign vmap.(vn){2}
for columns that map directly from a column of the data source.
6.2.2. Data Export Specifications
The default export()
method first calls the get_export_spec()
method to get a struct containing the specifications that define the details of the export process. This specification is then passed to export_table_values()
to export the data.
The export of data from a data model element back to the original data format is handled by the same variable map as the input, by default.
The init_export_data()
method is used to initialize the relevant output data structure before exporting to it, if the data_exists()
method returns false.